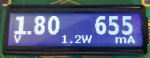
I tried to use the CFAG14432C as a display for a power supply. The code that I used came from the CFAG14432_SPI_Arduino example from this site.
When I call the function 'Send_FrameBuffer_To_LCD', an interference is shown on the display. The display is kept in graphics mode all the time.
Is this a known behaviour? Can we stop output to the display somehow during the update or is there any other way to get rid of this distortion?
I've tried extra 100nF capacitors on the power supply input connector and between GND and the contrast pin. That didn't make any difference.
Code:
void Send_FrameBuffer_To_LCD( void ) {
register uint8_t i;
register uint8_t j;
//
//Setting to graphics mode commented out, display is kept in graphics mode all the time.
//Commands were moved to the Arduino setup function
//
//write_command( 0x06 ); // entry mode -- cursor moves right, address counter increased by 1
//write_command( 0x34 ); // extended function
//write_command( 0x36 ); // graphic mode on
//
//Send the FrameBuffer RAM data to the LCD.
for( j = 0; j < FRAME_HEIGHT_PIXELS; j++ ) {
//Set Y address to this line, reset X address
Set_Graphics_Mode_Address( 0, j );
for( i = 0; i < FRAME_WIDTH_BYTES; i++ ) {
write_data( framebuffer[j][i] );
}
}
}
Looking for additional LCD resources? Check out our LCD blog for the latest developments in LCD technology.
Last edited: